Introduction
The Cloud Development Kit (CDK) is an IaC tool that is expected to be ran on a CI/CD environment. Although it can be successfully used on its own, the infrastructure that it creates is usually used in another step to “fully complete” a deployment.
In this post, we explore a way of parsing a CDK deployment’s output for further processing.
Our goal infrastructure
Let’s start by defining our example environment with our desired infrastructure:
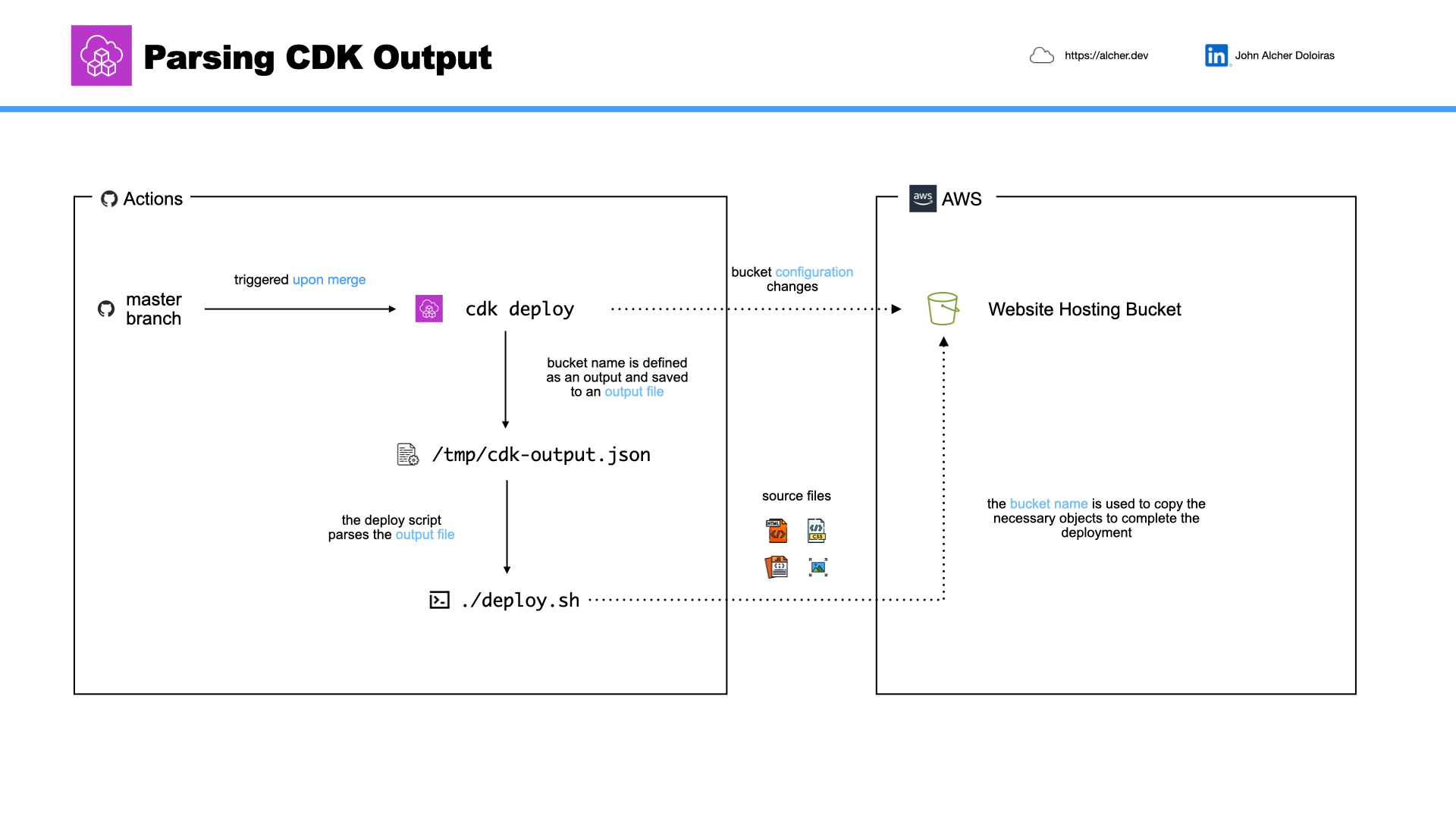
- A Github environment running Actions upon merging to
master
- An AWS environment containing an S3 Bucket for website hosting
- The Github Action calling
cdk deploy
to apply configuration changes - CDK dumping its output to an output file, containing the bucket name
- A separate deploy script that parses the output file, and copying objects to the S3 bucket to complete the deployment
Steps 1-3 are out of scope of this post and we’ll focus on how to implement steps 4 and 5.
Dumping CDK output to a file
CDK is commonly used in the following manner:
$ cdk synth
$ cdk deploy --require-approval never
In order for CDK to dump an output after deployment, we can use the CfnOutput
construct:
// cdk-stack.ts
const bucket = new s3.Bucket(this, 'WebsiteBucket');
new CfnOutput(this, 'WebsiteBucketName', {value: bucket.bucketName});
This will append to CDK’s output (to stdout
) the following information:
MyStack.WebsiteBucketName = my-bucket-name-qe0xj1532
We can parse this as-is by piping the deploy command to a parsing tool, but we can simplify it even more by defining an outputs-file
:
$ export CDK_OUTFILE=/tmp/cdk-output.json
$ cdk synth
$ cdk deploy --require-approval never --outputs-file $CDK_OUTFILE
The JSON file will look something like this:
{
"MyStack": {
"WebsiteBucketName": "my-bucket-name-qe0xj1532"
}
}
Parsing the output file
The output being formatted in a JSON file affords us a lot of convenience. We will use the jq
tool to help us parse this file so we can use the bucket name as our target deployment:
#!/usr/bin/env bash
CDK_OUTFILE=/tmp/out.json
SOURCE_DIR=./dist
DEST_BUCKET=$(jq -r '.MyStack.WebsiteBucketName' $CDK_OUTFILE)
aws s3 sync --delete "$SOURCE_DIR" s3://"$DEST_BUCKET"/
Conclusion
To get output from a CDK deployment into a JSON file, use the --outputs-file
flag. The JSON file can then be parsed by a tool like jq
for further processing.