Goal
The goal is to add a PostgreSQL container and an entrypoint script for the Django app that checks the DB’s availability before startup.
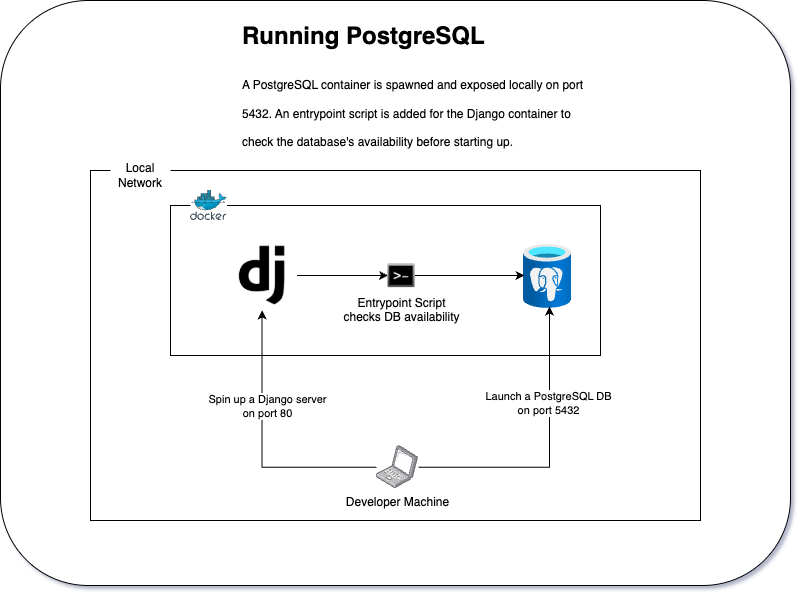
PostgreSQL Container
I added another service entry in my compose file for the PSQL database:
# docker-compose.yml
db:
image: postgres:17.4-bookworm
environment:
POSTGRES_DB: "lith_pg_db"
POSTGRES_USER: "lith_pg_user"
POSTGRES_PASSWORD: "lith_pg_password"
volumes:
- "./docker/data/postgres:/var/lib/postgresql/data"
ports:
- "5432:5432"
I prefer to keep the data volume in a gitignored directory, which in this case is docker/postgres
. An alternative option is to create an anonymous volume, but I prefer to visually see where the files go for easier inspection.
I set the DB credentials as environment variables, which requires changes to the Django settings file:
# src/config/settings.py
DATABASES = {
"default": {
"ENGINE": os.environ["DB_ENGINE"],
"NAME": os.environ["DB_DATABASE"],
"USER": os.environ["DB_USER"],
"PASSWORD": os.environ["DB_PASSWORD"],
"HOST": os.environ["DB_HOST"],
"PORT": os.environ["DB_PORT"],
},
}
These values are injected through the environment file:
# src/local.env
DB_ENGINE="django.db.backends.postgresql"
DB_DATABASE="lith_pg_db"
DB_USER="lith_pg_user"
DB_PASSWORD="lith_pg_password"
DB_HOST="db"
DB_PORT="5432"
Note: While I haven’t decided yet on how I’d structure my tests, I think it’s a good idea to make the DB_ENGINE changeable at runtime as to give me the option to switch to a different database when running tests (eg. sqlite)
Lastly, I’d need a Python database adapter in order to connect to Postgres. I’ve never had any issue with psycopg2-binary
, so I’d keep using this library.
$ echo psycopg2-binary >> src/requirements.in
Running make up
and make migrate
confirms the connectivity between Django and Postgres.
Entrypoint Script
Given enough time, Django’s startup routine will attempt to connect to Postgres before it’s ready to accept connections. Even using depends_on
in our compose file is not enough.
We can leverage Docker’s entrypoint option so we can confirm Postgres’ availability before starting the Django application.
# src/entrypoint.sh
if [ "$DB_ENGINE" = "django.db.backends.postgresql" ]
then
echo "Waiting for PostgreSQL..."
while ! nc -z $DB_HOST $DB_PORT; do
sleep 0.1
done
echo "PostgreSQL started"
fi
exec "$@"
Credit to testdriven.io’s article for the entrypoint script idea.
A couple of changes are required in the application’s Dockerfile:
- As
nc
(netcat) is not installed by default in Debian, we’ll have to install it. - Point to the entrypoint script using the
ENTRYPOINT
directive. - I also took the time to explicitly use the
bookworm
version of Python
# src/Dockerfile
FROM python:3.13-bookworm
# ...snip
RUN apt update && apt -y dist-upgrade
RUN apt install -y netcat-traditional
# ...snip
ENTRYPOINT ["/app/entrypoint.sh"]
Running make up
should now consistently spin up the application and db containers successfully.
Conclusion
In this lab, I added a PostgreSQL container and made changes to the Django container so it can successfully connect to the database. I also utilized an entrypoint script so the application waits for the database to be ready, preventing any failures where the application started too early.
The source is available at the feature/003-postgresql
branch.