Goal
The goal is to be able to start a dockerized Django server for local development, with accompanying quality tools for linting, formatting, testing, and Django management.
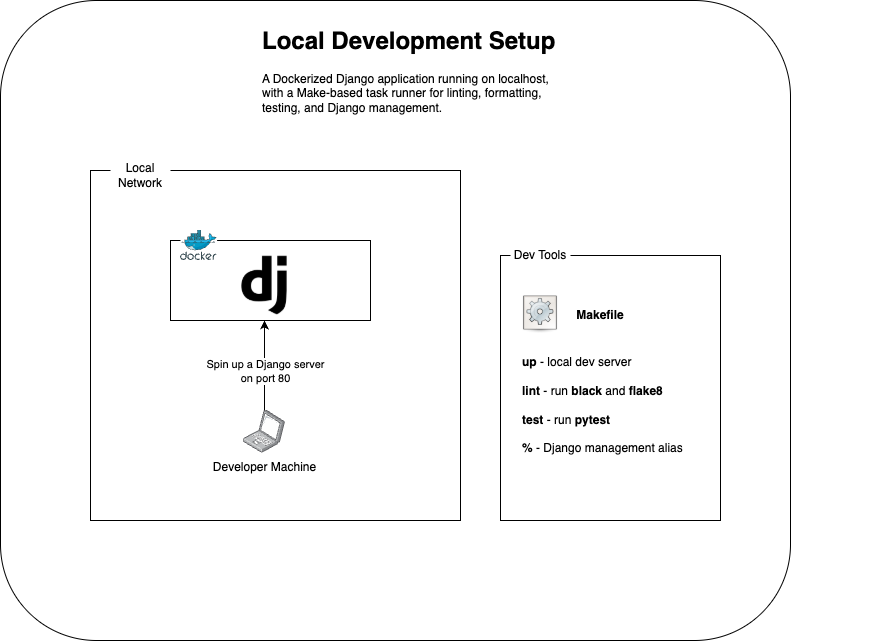
Local Django server
I started by creating a src
directory and creating a once-off virtual environment to install Django into in order to run the startproject
command. I used a personal convention of naming the project config
and targeting the current directory (which is src
) as its output.
$ mkdir src && cd src
$ python -m venv venv
$ source ./venv/bin/activate
$ pip install Django
$ django-admin startproject config .
Before I forget, I added Django
as a project requirement by creating a requirements.in
file.
$ echo Django >> requirements.in
I’d want to be able to pin the dependency versions, but I’ll tackle that in the future where I’d generate a requirements.txt
file from the requirements.in
file with locked versions (a-la package-lock.json
in the npm
world). But this should suffice for now.
To keep things tidy, I removed all the comments in the generated Python files and replaced all double quotes into single quotes. sed
would’ve been fine for this, but I used PyCharm’s Find-and-Replace function.
We can test the Django server by running the runserver
command.
$ python manage.py runserver 0.0.0.0:80
Dockerize the Django app
I created a very basic python Dockerfile
that installs the project dependencies:
# src/Dockerfile
FROM python:3.13
WORKDIR /app
ENV PYTHONDONTWRITEBYTECODE=1
ENV PYTHONUNBUFFERED=1
RUN pip install --upgrade pip
COPY requirements.in .
RUN pip install --no-cache-dir -r requirements.in
COPY . .
I made changes to the src/config/settings.py
so that the default SECRET_KEY
, DEBUG
, and ALLOWED_HOSTS
are loaded from the environment and not populated directly.
SECRET_KEY = os.environ["SECRET_KEY"]
DEBUG = int(os.environ["DEBUG"])
ALLOWED_HOSTS = os.environ["ALLOWED_HOSTS"].split(" ")
Note: I intentionally accessed
os.environ
via dictionary keys rather than using.get
with a default value. I’d rather the app blow up in startup when it’s misconfigured rather than it making configuration assumptions.
For local development, I stored the expected values in the src/local.env
file:
SECRET_KEY="dev-key"
DEBUG="1"
ALLOWED_HOSTS="localhost 0.0.0.0"
This file is consumed in the docker-compose.yml
file which starts up the application container on port 80, while also binding the host src
directory to the container’s workdir for smooth local development:
services:
app:
build: src
command: [ "python", "manage.py", "runserver", "0.0.0.0:80" ]
ports:
- "80:80"
env_file:
- src/local.env
volumes:
- "./src:/app"
The container can be spun up using the docker-compose’s up
command
$ docker compose up --build
Dev Tools
I decided on black
and flake8
as my code linting/formatting tools. They’re mostly personal preference (any color as long as it’s black kind of thing) that had worked for me. It’s an annoyance that each tool have a separate configuration file, particularly how flake8
doesn’t work with pyproject.toml
when most tools does already. But it does the job reasonably well.
My configuration is nothing special — just ignoring a handful of rules for black
+ flake8
compatibility and increasing the line length to 100.
# src/pyproject.toml
[tool.black]
line-length = 100
# src/tox.ini
[flake8]
max-line-length = 100
ignore =
E501,
E203,
E701,
W503,
Adding them to the requirements file and rebuilding the container should install them. They can then be run via docker-compose’s exec
command:
$ echo black >> src/requirements.in
$ echo flake8 >> src/requirements.in
$ docker compose up --build
$ docker compose exec app black .
$ docker compose exec app flake8 .
For more information, here’s a handy guide from Lj Miranda on how to configure them for pre-commit hooks.
As for tests, pytest
is still the de-facto library. I also pulled in pytest-django
to make it easier to run Django test cases with pytest
.
$ echo pytest >> src/requirements.in
$ echo pytest-django >> src/requirements.in
I added an entry in the tox.ini
file for the pytest-django
configuration.
[pytest]
DJANGO_SETTINGS_MODULE = config.settings
python_files = *_test.py
Note: using the
*_test.py
pattern for test files is a personal preference that I got from Golang’s test runner. I also follow the “test lives with the source” approach wherefoo.py
andfoo_test.py
are in the same directory.
I then created a new Django lith
app where I’d house all the Django code. I’m not a fan of multiple applications if they’re not specifically built for publication.
$ docker compose exec app python manage.py startapp lith
As with the earlier housekeeping, I deleted all files that are not used. I then created a simple view and an accompanying test:
# src/lith/views.py
from django.http import HttpRequest, JsonResponse, HttpResponse
def home(request: HttpRequest) -> HttpResponse:
return JsonResponse({"message": "hello, world"})
# src/lith/views_test.py
import json
from django.test import RequestFactory
from .views import home
def test_hello_world():
factory = RequestFactory()
request = factory.get("/")
response = home(request)
assert 200 == response.status_code
json_response = json.loads(response.content)
assert "hello, world" == json_response["message"]
Running pytest
confirms that the setup succeeds:
$ docker compose exec app pytest
Makefile
I chose Make as a very basic task runner. I know this isn’t exactly what the tool is designed for, but it does its job and its prevalence (both in terms of availability in most systems and developer familiarity) is hard to beat.
I created targets for spinning up the containers, running linting/formatting, running tests, and a catch-all alias for running Django management commands.
up:
docker compose up --build
lint:
docker compose exec app black .
docker compose exec app flake8 .
test:
docker compose exec app pytest
# Maps the target to a Django management command.
# ex: make migrate -> python manage.py migrate
%:
docker compose exec app python manage.py $*
Conclusion
In this lab, I build the scaffold for local development of a Dockerized Django application.
The source is available at the feature/001-local-development branch
.